stack은 후입선출(LIFO)의 자료 구조이다. 즉, 마지막에 넣은 데이터가 가장 먼저 꺼내진다.
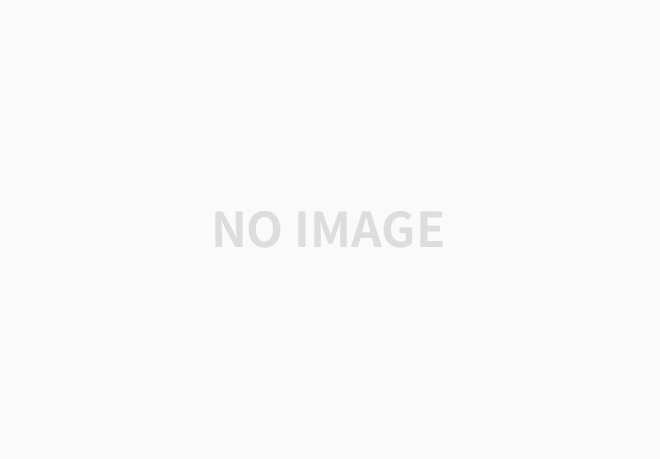
[구현하기]
class Stack<T> {
private stack: Array<T> = [];
private max = 0;
private len = 0;
constructor(max: number) {
this.max = max;
}
top(): T {
return this.stack[this.len - 1];
}
pop(): Stack<T> {
if (this.len === 0) throw new Error('stack is empty');
this.stack.splice(this.len - 1, 1);
this.len--;
return this;
}
push(value: T): Stack<T> {
if (this.len === this.max) throw new Error('stack is full');
this.stack[this.len] = value;
this.len++;
return this;
}
empty(): boolean {
return this.stack.length === 0 ? true : false;
}
get size(): number {
return this.max;
}
}
[사용하기]
const myStack = new Stack<number>(10);
console.log(myStack.empty());
myStack.push(1);
myStack.push(2);
myStack.push(3);
myStack.push(4);
console.log(myStack);
myStack.pop();
myStack.pop();
myStack.pop();
console.log(myStack);
console.log(myStack.top());
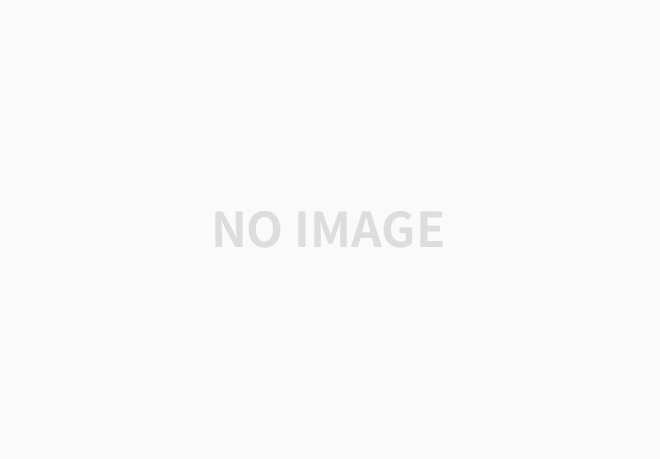
상자 안에 내용물을 쌓아둔다고 생각하면 이해하기 더 쉬워진다.
'갬발자의 프로그래밍 > 알고리즘 & 자료구조' 카테고리의 다른 글
[자료구조] Queue 구현하기 (0) | 2021.03.31 |
---|---|
[자료구조] Linked List 직접 구현하기. (0) | 2021.03.30 |
큐 와 스택 (0) | 2020.02.18 |
BIG-O 표기 (0) | 2020.02.14 |
댓글